Introducing the Eventualist.Extension Package
While programming some other projects, I came upon recurring tasks which causes me to produce a lot of boilerplate code. That is why I started the Eventualist.Extension package (Eventualist is a now defunct project I was working on). It contains many handy extension methods and even one completely new class to make my, and your life easier easier.
Installation instructions
More information about this package can be found here. The easiest way to install this package in Visual Studio 2022, is right click the project or the solutions and choose ‘Manage Nuget Packages’ or ‘Manage Nuget Packages For Solution’ and you will see this screen:
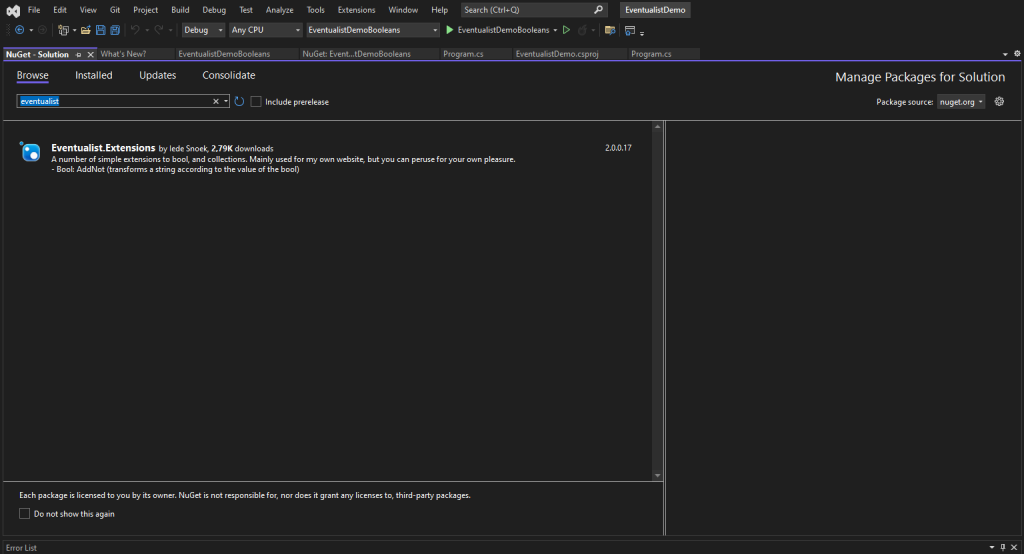
As you can see, typing ‘Eventualist’ in the searchbox will bring up this package. Now simply click the ‘Install’ button
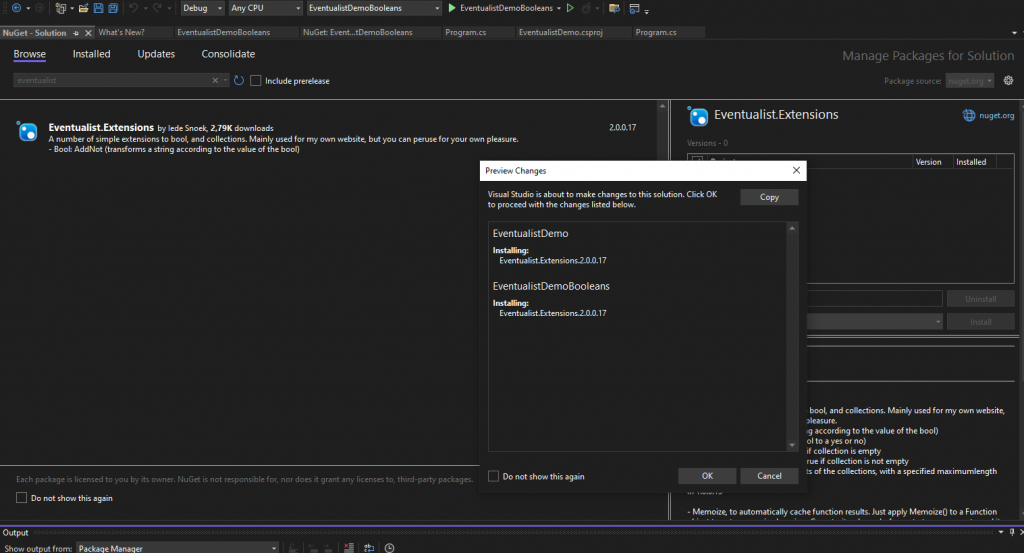
Now click ‘OK’ and the package will be installed in your project or your solution.
Important: please use version 2.0.0.17 of this package, as there was an error in the Abbreviate extension method.
Collections
IsEmpty and IsNotEmpty
This is simply a wrapper for the LINQ Any function. The use is quite straightforward:
using Eventualist.Extensions.Collections;
List<string> emptyList = new();
List<string> testList = new List<string>()
{
"One",
"Two",
"Three",
"Four",
"Five",
"Six",
"Seven",
"Eight",
"Nine",
"Ten",
"Eleven"
};
Console.WriteLine(emptyList.IsEmpty() ? "List is empty" : "List is not empty");
Console.WriteLine(testList.IsNotEmpty()? "TestList is not empty": "TestList is empty");
CreateOrderedString
This extension is basically a combination of the OrderBy operator and the string.Join method.
It can be used as follows:
using Eventualist.Extensions.Collections;
List<string> emptyList = new();
List<string> testList = new List<string>()
{
"One",
"Two",
"Three",
"Four",
"Five",
"Six",
"Seven",
"Eight",
"Nine",
"Ten",
"Eleven"
};
var numberString = testList.CreateOrderedString(x => x);
Console.WriteLine(numberString);
This will output the following string: Eight,Eleven,Five,Four,Nine,One,Seven,Six,Ten,Three,Two
You can specify the key selector i.e. the property which should be sorted, and the separator. The separator defaults to ‘,’.
For now the ordering is only ascending.
Divide
This is a handy function, it divides a list into sublists of a specified length, which defaults to 3. So, in case of 11 items, and groupsize 3, I will get 3 lists of 3 items, and one of 2. To demonstrate this, try the following code:
using Eventualist.Extensions.Collections;
List<string> testList = new List<string>()
{
"One",
"Two",
"Three",
"Four",
"Five",
"Six",
"Seven",
"Eight",
"Nine",
"Ten",
"Eleven"
};
var subLists=testList.Divide(3);
foreach (var list in subLists)
{
var listString = string.Join(',', list);
Console.WriteLine(listString);
}
If you specifiy a groupsize of zero, an exception is thrown.
ExtendedDictionary
ExtendedDictionary is a class which inherites from Dictionary and can be used in much the same way. There are two differences however, let’s look at this code:
using Eventualist.Extensions.Collections;
ExtendedDictionary<string,string> testDictionary = new ExtendedDictionary<string, string>
{
{"A","First letter"},
{"B","Second Letter"},
{"C","Third letter"}
};
var getWithSpecifiedDefaultValueResult = testDictionary["D","Unknown key"];
Console.WriteLine($"The default value is {getWithSpecifiedDefaultValueResult}");
var getWithDefaultValueResult = testDictionary["D"];
Console.WriteLine($"Default value is {getWithDefaultValueResult}");
If the key exists in the dictionary, ExtendedDictionary behaves like an ordinary dictionary.
However, as you can see, if the key does not exists, you have to options:
- Specify a default value, like “Unknown key” in the example
- Not specifying a default and getting the default value, in the case of the example default(string) which is null.
Functions
Memoize converts Func objects into cached Func objects by inserting a caching mechanism, this can reduce the time taken for some operations. An example here:
using Eventualist.Extensions.Functions;
var sumclass = new SumClass();
var sumMethod = ((int a, int b) => sumclass.add(a, b));
var memoizedSumMethod = sumMethod.Memoize();
var test = memoizedSumMethod(2, 3);
Console.WriteLine($"Test is {test}");
class SumClass
{
public int add(int a, int b)
{
return a + b;
}
}
At this moment it supports methods of up to six parameters. This might be extended in the future. Also support for async functions might bve added
Strings
ShowIfNone
This converts a string to “None” or another specified text, if the string is null or empty, otherwise the string will be returned.
using Eventualist.Extensions.Strings;
string? emptyString = null;
Console.WriteLine(emptyString.ShowIfNone());
Abbreviate
This extension method can break off long messages and replace the rest with an abbreviationsymbol which defaults to ‘…’.
using Eventualist.Extensions.Strings;
string testString = "The quick brown fox jumps over the lazy dog and back again";
var abbreviatedString = testString.Abbreviate(12);
Console.WriteLine(abbreviatedString);
This will print: The quick brown …
This can be very handy in web pages and on some mobile apps. As you can see, abbreviations occur on word-borders, not in the middle of words.
Booleans
We start with some utility methods for booleans:
First there is the ‘AddNot’ method to add a negation to a word when needed:
using Eventualist.Extensions.Booleans;
bool isEnabled = false;
var enabledString = isEnabled.AddNot("enabled");
Console.WriteLine($"The switch is {enabledString}");
This will print “The switch is not enabled”
Then there is the ToYesOrNoMethod, which transforms a boolean to a “Yes” or “No”, or to the localized variant, “Yes” and “No” are default values:
using Eventualist.Extensions.Booleans;
bool isEnabled = false;
var enabledString = isEnabled.ToYesOrNo();
Console.WriteLine($"The switch is enabled: {enabledString}");
This should print out “The switch is enabled: no”.